Day 04: Pre-Class Assignment: Advanced Git Concepts - Forking, Branching, and Merging#

Student Identification#
Please do not modify the structure or format of this cell. Follow these steps:
Look for the “YOUR SUBMISSION” section below
Replace
[Your Name Here]
with your nameIf you worked with groupmates, include their names after your name, separated by commas
Do not modify any other part of this cell
Examples:
Single student: John Smith
Group work: John Smith, Jane Doe, Alex Johnson
YOUR SUBMISSION (edit this line only):
✅ [Your Name Here]
Note:
Keep the “✅” symbol at the start of your submission line
Use commas to separate multiple names
Spell names exactly as they appear in the course roster
This cell has been tagged as “names” - do not modify or remove this tag
Table of Contents#
Learning Goals#
By completing this pre-class assignment, you will:
Review basic Git concepts and commands.
Understand the concepts of forking and branching in Git.
Learn how to fork a repository on GitHub.
Practice creating and managing branches.
Understand the differences between forking and branching.
Practice merging branches in your own repository.
Prepare for collaborative coding using these Git features.
Git Review and Advanced Concepts#
Quick Review of Basic Git Concepts:#
Repository: A container for your project, including all files and their version history.
Commit: A snapshot of your repository at a specific point in time.
Push: Upload local repository content to a remote repository.
Pull: Fetch and merge changes from a remote repository to your local repository.
Forking#
Forking is the process of creating a personal copy of someone else’s project. Forks act as a bridge between the original repository and your personal copy. You can freely experiment with changes without affecting the original project.
Key points about forking:
It’s done on GitHub (or similar platforms), not with Git commands.
It allows you to propose changes to someone else’s project.
It’s often used in open-source contributions.
Branching#
Branching allows you to diverge from the main line of development and work on different features or ideas in isolation.
Key Git commands for branching:
git branch
: List, create, or delete branches.git checkout <branch_name>
: Switch to an existing branch.Adding the
-b
flag (i.e.,git checkout -b <new_branch_name>
) creates a new branch and switches to it in one command.
git merge <branch_name>
: Merge changes from one branch into your current branch.
Merging#
Merging is the process of combining changes from different branches. It’s a crucial part of the Git workflow, allowing you to integrate work from different lines of development.
Key points about merging:
It’s typically done to combine feature branches back into the main branch.
Merging can sometimes result in conflicts that need to be resolved manually.
The
git merge
command is used to perform merges.
Differences between Forking, Branching, and Cloning#
Aspect |
Forking |
Branching |
Cloning |
---|---|---|---|
Definition |
Creating a personal copy of someone else’s project on a server |
Creating a separate line of development within a repository |
Creating a local copy of a repository |
Command |
Done through GitHub interface |
|
|
Scope |
Creates a new repository |
Works within an existing repository |
Creates a full local copy of a repository |
Typical Use Case |
Contributing to projects you don’t have write access to |
Developing features or fixing bugs within your own project |
Getting a local copy of a repository to work on |
Relationship to Original |
Separate but linked through GitHub |
Part of the same repository |
Independent copy, can be kept in sync |
Collaboration |
Allows for pull requests to the original project |
Allows for merging within the same repository |
Allows for pushing changes if you have access |
Visibility |
Public (usually) |
Private to the repository |
Local to your machine |
Key points:
Forking is typically used for open-source contributions or starting your own project based on someone else’s.
Branching is used for parallel development within a project you have access to.
Cloning is used to get a local copy of a repository, whether it’s your fork, someone else’s project, or a project you’re contributing to.
You often use these in combination: fork a repository on GitHub, clone your fork to your local machine, then create branches for specific features or fixes.
🗒️ Task: Explain in your own words (2-3 sentences) when you would choose to fork a repository versus creating a new branch. Provide an example.
✏️ Answer:
Hands-on Practice#
Let’s practice forking, branching, and merging using a repository prepared for this class:
Part 1: Forking and Branching#
Fork the repository:
cmse802-s25-git_testing
to your GitHub account:Go to the repository page and click the “Fork” button in the top-right corner.
Clone your forked repository in the
CMSE802/repositories
directory on your local machine:Create and switch to a new branch with a name of your choice:
git checkout -b <new_feature_branch_name>
Replace
<new_feature_branch_name>
with a name of your choice, e.g.,add-john-doe
.Open the README.md file in a text editor and add your name and last name to the list of contributors.
Commit your changes:
git add README.md git commit -m "Added [Your Name] to contributors list"
Push your changes to your fork:
git push origin <new_feature_branch_name>
Go to your fork on GitHub and create a pull request from your
<new_feature_branch_name>
to the main branch of the originalcmse802_git_testing
repository. Your pull request counts towards the grade for this assignment.
Part 2: Branching and Merging in Your Class Repository#
Now, let’s practice branching and merging in your existing class assignment repository:
Clone your class assignment repository
cmse802-S25-turnin
on your local machine (if you haven’t already):git clone https://github.com/YOUR-USERNAME/cmse802-S25-turnin.git cd cmse802-S25-turnin
Create a new branch for a hypothetical feature:
git checkout -b new-feature
Make a new directory called
PreClassAssignments
and add a new file namedgit_forking_branching_merging.md
with the following content:# New Feature: Advanced Git Concepts This file demonstrates the process of forking, branching, and merging in Git. ## Steps to Merge a New Feature 1. Create a new branch for the feature. 2. Make changes to the code or documentation. 3. Commit your changes. 4. Push the new branch to your remote repository. 5. Merge the new feature branch into the main branch. 6. Push the merged changes to your remote repository.
Commit your changes:
git add . git commit -m "Added new file for advanced Git concepts"
Push this new branch to your remote repository:
git push origin new-feature
Switch back to your main branch:
git checkout main
Merge the new-feature branch into main:
git merge new-feature
Push the merged changes to your remote repository:
git push origin main
Reflection#
Consider the following questions:
How do you think forking, branching, and merging contribute to efficient collaboration in software development?
What challenges do you anticipate when working with forks and branches in a team setting?
How might these
git
features be useful in your future computational modeling projects?Can you think of a scenario where merging might lead to conflicts? How would you approach resolving such conflicts?
🗒️ Task: Write a short paragraph (5-6 sentences) reflecting on the questions above.
✏️ Answer:
Review (Optional): Using solve_ivp
for Solving Differential Equations#
Before we dive into implementing our models in the upcoming in-class project, let’s review how to use solve_ivp
from SciPy to solve systems of ordinary differential equations (ODEs). This function will be a crucial tool for simulating the dynamics of the models you’ll be working with during the in-class assignment.
If you feel comfortable with solve_ivp
, feel free to skip this section.
What is solve_ivp?#
solve_ivp
is a function from SciPy’s integrate module that numerically integrates a system of first-order ODEs. It’s particularly useful for solving initial value problems, which is exactly what we’ll be doing with our models in the class project.
Basic Usage#
Here’s the basic structure of using solve_ivp
:
from scipy.integrate import solve_ivp
def model(t, y, param1, param2):
# Define your system of ODEs here
dy_dt = [...] # List of derivatives
return dy_dt
# Set up parameters and initial conditions
t_span = (t_start, t_end)
y0 = [initial_value1, initial_value2, ...]
params = (param1_value, param2_value)
# Solve the ODE
solution = solve_ivp(
fun = model, # Function containing the ODEs
t_span = t_span, # Tuple with the start and end times
y0 = y0, # Initial conditions
args=params # Other parameters needed by the model. Notice that this is a tuple!
)
Key Parameters#
fun
: The function defining the ODE system (ourmodel
function).t_span
: A tuple (t0, tf) giving the interval of integration.y0
: Initial state (list or array).args
: Additional arguments to pass to the model function.method
: The integration method to use (default is ‘RK45’, but others are available).
Accessing the Solution#
After solving, you can access:
solution.t
: Array of time points.solution.y
: Array of solution values. Each row corresponds to a variable, each column to a time point.
Example: Simple Harmonic Oscillator#
Let’s look at a simple example to illustrate the usage. The equations of an harmonic oscillator are
These are the equations to be written in the function harmonic_oscillator
.
Run the code cell below to see solve_ivp
in action for a harmonic oscillator system:
import numpy as np
from scipy.integrate import solve_ivp
import matplotlib.pyplot as plt
def harmonic_oscillator(t, y, k, m):
"""Simple harmonic oscillator ODE
Parameters
----------
t : float
Time
y : list
List containing the displacement and velocity
k : float
Spring constant
m : float
Mass
Returns
-------
list
List containing the velocity and acceleration
"""
x, v = y
dxdt = v
dvdt = -k/m * x
return [dxdt, dvdt]
# Parameters
k = 1.0 # spring constant
m = 1.0 # mass
# Initial conditions
y0 = [1.0, 0.0] # initial displacement and velocity
# Time span
t_span = (0, 10)
# Solve ODE
sol = solve_ivp(harmonic_oscillator, t_span, y0, args=(k, m), dense_output=True)
# Generate smooth solution curve
t = np.linspace(0, 10, 200)
y = sol.sol(t)
# Plot results
plt.plot(t, y[0])
plt.xlabel('Time')
plt.ylabel('Displacement')
plt.title('Simple Harmonic Oscillator')
plt.grid(True)
plt.show()
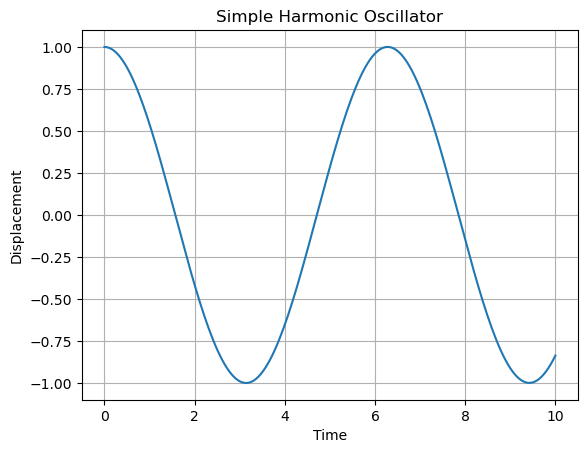
This example demonstrates how to set up the ODE function, solve it using solve_ivp
, and plot the results.
As you implement your chosen model, you’ll follow a similar structure, defining your system of ODEs and using solve_ivp
to solve them over time.
Assignment wrap-up#
Please fill out the form that appears when you run the code below. You must completely fill this out in order to receive credit for the assignment! If running the cell doesn’t work in VS Code copy the link src
and paste in the browser. Make sure to sign in with your MSU email.
from IPython.display import HTML
HTML(
'''
<iframe
src="https://forms.office.com/r/AEc6LS6xKF"
width="800px"
height="600px"
frameborder="0"
marginheight="0"
marginwidth="0">
Loading...
</iframe>
'''
)
Congratulations, you’re done with your pre-class assignment!#
Now, you just need to submit this assignment by uploading it to the course Desire2Learn web page for the appropriate pre-class submission folder. (Don’t forget to add your name in the first cell).
© Copyright 2024, Department of Computational Mathematics, Science and Engineering at Michigan State University